Domain Driven Design for Android Developers
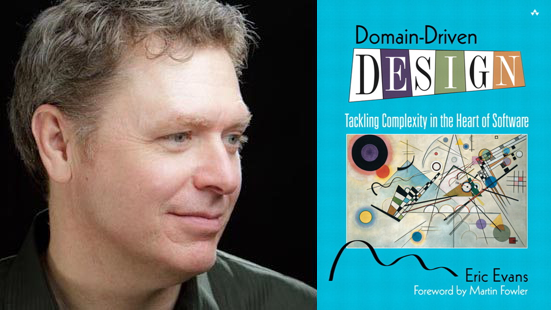
I was exposed to Domain Driven Design in 2014 or so, almost 10 years after the book was written by Eric Evans, on one of the major products I was working on back then. I’ve found it quite beneficial for figuring out the problem domain and writing maintainable Android apps.
What is Domain Driven Design?
Domain Driven Design is a framework or a theoretical workflow for approaching complex software projects by effectively modelling the real world in code and using the inherent power of OOP to encapsulate the business logic of the domain.
Let’s take a look at some of the principles that the book talks about.
No Ambiguous Modelling: use a ubiquitous language
An important part of DDD is a set of patterns and common terminology between the business/domain experts and the development team. Referred to as the Ubiquitous Language, it captures the business domain of the application and allows everyone on the project to talk about it using a shared vocabulary.
For example, when designing e-commerce software, SKU should mean the same to both the business and code. Modelling Order and LineItems in code should reflect the domain well.
I remember writing code, naming the same concept differently at different locations. Something like Item when adding to cart and Products while placing the order. This created a lot of confusion 6 months later while adding features while it made “perfect” sense when I wrote it. 🤦
Figure out all Bounded Contexts
Ideally, it would be preferable to have a single, unified model. While this is a noble goal, in reality, it typically fragments into multiple models.
Explicitly define the context within which a model applies. Explicitly set boundaries in terms of team organization, usage within specific parts of the application, and manifestations such as code bases and database schemas. Keep the model strictly consistent within these bounds.
Example: In an e-commerce system it could be search, ordering, fulfilment, tracking, etc.
This could also help you in identifying modules in your app. As you see, packaging by feature creates natural bounded contexts.
Define Entities
Entity is an object that is not defined by its attributes, but rather by its identity. These are domain-level objects and works well when defined similarly on the server and client.
Example: In an airline app it could be a Seat. Most airlines distinguish each seat uniquely on every flight. Each seat is an entity in this context.
Define Value Objects
An object that contains attributes but has no conceptual identity. They should be treated as immutable.
Example: Objects that represent Money or DateRange
Identify Aggregates
A collection of objects that are bound together by a root entity, otherwise known as an aggregate root. The aggregate root guarantees the consistency of changes being made within the aggregate boundary by forbidding external objects from holding references to its members.
Example: Customer is an aggregate of several other entities like Address, Orders, etc.
Define Services
When an operation does not conceptually belong to any object, like retrieval of specified information or the execution of a set of operations, you can implement these operations in services. This is also called service-orientation in the context of software architecture.
Example: AuthenticationService for login or signup.
Define Repositories
Methods for retrieving domain objects should delegate to a specialized Repository object such that alternative storage implementations may be easily interchanged. This is also called the Repository pattern in software architecture. This could be an in-memory cache, disk or the network.
Example: UserStore to save and retrieve details of a User.
Practicing DDD
As you may have noticed, if you are a fairly well experienced with Object Orientated Programming, you might already be familiar with a lot of patterns and terminologies of DDD. The biggest takeaway for me from this book was that non-technical members of the team should understand the code that is written, and all technical members of the team should fully understand the inner workings of the business/domain. This helps in making the inevitable refactoring process easier even when the team working on the software changes.
DDD does not depend on any tool and is more of a workflow for domain modelling. It is difficult to get it right the first time. DDD needs room to evolve and relies on iterative refactoring for achieving the best possible representation of the domain.
So the next time you start writing your app, get your developers, designers, product managers and projects managers together and apply these principles.
References
- Definitions on Wikipedia.
- DDD review by Philip Brown.