Android Gotchas: #2 View.GONE layouts
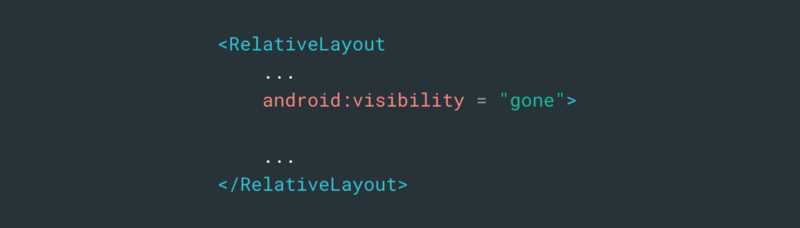
Toggling Visibility
I’ve always had to deal with layouts that are GONE
by default and are made VISIBLE
based on certain conditions.
<RelativeLayout
...
android:visibility="gone">
</RelativeLayout>
In most cases this isn’t a simple View
, but a complex ViewGroup
. This could be something like a fancy progress indicator or details that show on click of a button.
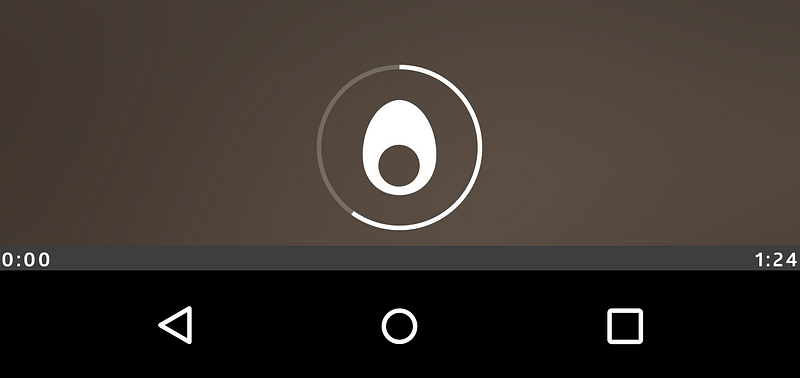
The views participate in the layout inflation even if they are GONE
by default. If you have many views like these in a complex layout, it might slow down rendering and increase memory usage.
Rescue time
Lazy load resources at runtime only when they are needed by:
1. Creating a ViewStub
<ViewStub
android:id="@+id/stub”
...
android:inflatedId="@+id/loading_progress"
android:layout="@layout/progress_overlay"/>
A ViewStub
is an invisible, zero-sized, no dimension View
that doesn’t draw anything or participate in the layout.
2. Loading the ViewStub
val stub = findViewById(R.id.stub) as ViewStub;
stub.inflate();
// or
// stub.visibility = View.VISIBLE;
When a ViewStub
is made visible
, or when inflate()
is invoked, the layout resource is inflated. The ViewStub
then replaces itself in its parent with the inflated layout. The ID of the inflated layout is the one specified by the android:inflatedId
attribute of the ViewStub
.
“It’s cheap to inflate and cheap to leave in a view hierarchy.”
Boom 💥